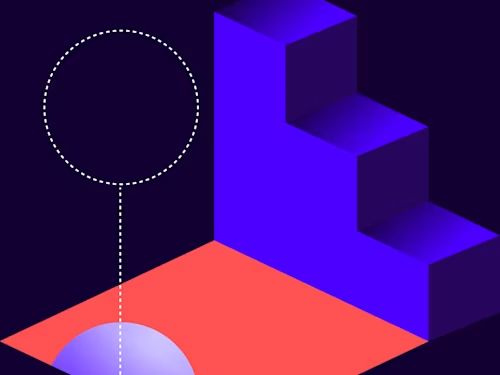
Configuring Salesforce for scalable and secure Docusign integrations
For integrating Docusign with Salesforce, you're not limited to the Apex Toolkit. Configuring Salesforce Named Credentials and OpenID Connect provides a robust alternative, enabling enhanced control, scalability, and security for your integrations.

Integrating Docusign with Salesforce is a powerful way to streamline document management and e-signature workflows. While the Docusign Apex Toolkit offers a convenient starting point, its limitations, such as dependency on managed packages and restricted flexibility, can pose challenges for advanced use cases. Fortunately, leveraging the Docusign REST API with Salesforce Named Credentials and OpenID Connect provides a robust alternative, enabling enhanced control, scalability, and security for your integrations.
In this blog, I’ll guide you through setting up and using these tools to bypass Apex Toolkit constraints. You’ll learn how to:
Configure Salesforce Named Credentials for seamless REST API communication.
Use OpenID Connect to establish a secure and scalable authentication flow.
Leverage the Docusign REST API for custom e-signature workflows.
By the end, you'll understand how to build a more flexible and efficient integration that meets your unique business requirements. Whether you’re a developer seeking advanced customisations or an admin aiming to optimise processes, this guide is for you!
To set up OpenID Connect (OIDC) as an Identity Provider for DocuSign API authentication in Salesforce, configure an External Credential with an OpenID Connect authentication provider. Docusign supports OIDC, and this approach enables Salesforce to manage identity verification with enhanced security. Follow these steps to configure OpenID Connect with DocuSign as the identity provider.
Step 1: Set up a Docusign integration key
Log in to your Docusign developer account.
Go to Admin > Integrations > Apps and Keys.
Create a New App:
Select Add App and Integration Key.
Enter a name for the app.
Save.
Copy the integration key (client ID).
Generate the client secret:
Select Actions > Edit > Add Secret key.
Copy the secret key.
Configure a redirect URI:
Add a redirect URI specifically for Salesforce’s OpenID Connect. It should be in the format:
For the production environment: https://login.salesforce.com/services/authcallback/your-app-name
For the demo environment: https://test.salesforce.com/services/authcallback/your-app-name
Save.
Step 2: Configure OpenID Connect as an authentication provider in Salesforce
Go to Setup in Salesforce.
Search for and select Auth. Providers.
Create a new authentication provider:
Provider Type: Select Open ID Connect.
Name: Choose a descriptive name, such as Docusign.
URL Suffix: Docusign.
Consumer Key: Enter the Client ID from your DocuSign app.
Consumer Secret: Enter the Client Secret from your DocuSign app.
Authorize Endpoint URL: Use https://account.docusign.com/oauth/auth (for production) or https://account-d.docusign.com/oauth/auth for demo).
Token Endpoint URL: Use https://account.docusign.com/oauth/token (for production) or https://account-d.docusign.com/oauth/token for demo).
User Info Endpoint URL: Use https://account.docusign.com/oauth/userinfo
Default Scopes: Set to signature extended.
Select Send access token in the header.
Select Include Consumer Secret in SOAP API Responses.
Save. This saves your auth provider and generates Salesforce configuration URLs.
Copy the callback URL.
From your Docusign account, navigate to Admin > Integrations > Apps and Keys.
From the Actions menu for your integration key, select Edit.
For Redirect URIs, paste the callback URL.
Save.
Step 3: Create an external credential in Salesforce
Go to Setup, search for Named Credentials, and then select External Credentials.
Create a new external credential with the following details:
Label: DocusignExternalCredential
Name: DocusignExternalCredential
Authentication Protocol: OAuth 2.0
Authentication Flow Type: Browser Flow.
Scope: signature extended
Authentication Provider: Select the OpenID Connect authentication provider you created in Step 2 above.
Save the external credential.
Create principals.
Scroll down to Principals.
To create a principal for the external credential, select New or select Edit from the Actions menu of an existing principal.
Enter the information for the principal:
Parameter Name: DocusignNamedPrincipal
Identity Type: Choose either Named Principal or Per User Principal. You can set up each external credential to use an org-wide named principal or per-user authentication. A named principal applies the same credential or authentication configuration for the entire org, while per-user authentication provides access control at the individual user level.
Scope: signature extended
Save the principal.
Create a permission set for external credential principal access.
From Setup, in the Quick Find box, enter Permission Sets, and then select Permission Sets.
Select New.
Enter your permission set name: ExternalCredentialPermission
Save your changes.
Select External Credential Principal Access.
Select Edit.
Move the external credential principal (example: DocusignNamedPrincipal) from the Available to the Enabled column.
Save your changes.
Step 4: Create a named credential linked to the external credential
In Setup, search for and select Named Credentials.
Create a new named credential:
Label: Enter a descriptive label, such as DocusignAPI.
Name: This name will be used in Apex.
URL: Enter the Docusign API base URI: https://demo.docusign.net/restapi/v2.1 (sandbox) or https://www.docusign.net/restapi/v2.1 (production). Note: Your production base URI may differ. Please check your Apps and Keys page.
Certificate: Leave blank unless you have specific certificate requirements.
External Credential: The name of an external credential. Link it to the external credential created in Step 3.
Save.
Step 5: Use named credentials in Apex
Reference the named credential:
In your Apex code, use the name of your Named Credential in a callout to call the Docusign REST API.
Example: Apex code to change the email subject and message of a draft envelope. This uses the Envelopes: update method of the eSignature REST API.
String accountId = '2ec17bee-90f1-xxxx-af85-b1ee3558f9xx'; String envelopeId = '7c8b166e-37f0-xxxx-b3c8-f80fc5865cxx'; // Create a new http object to send the request object HttpRequest req = new HttpRequest(); // set endpoit to callout:My_Named_Credential/some_path req.setEndpoint('callout:DocusignAPI/accounts/'+accountId+'/envelopes/'+envelopeId); req.setMethod('PUT'); req.setHeader('Content-Type', 'application/json'); // Construct the payload Map<String, Object> envelopeUpdate = new Map<String, Object>(); envelopeUpdate.put('emailSubject', 'new email subject'); envelopeUpdate.put('emailBlurb', 'new email message'); // Convert the payload to JSON String requestBody = JSON.serialize(envelopeUpdate); req.setBody(requestBody); Http http = new Http(); HttpResponse res = http.send(req); if (res.getStatusCode() == 200) { System.debug('Envelope sent successfully: ' + res.getBody()); } else { System.debug('Error: ' + res.getBody()); }
Example: Apex code to update a recipient. This uses the EnvelopeRecipients: update method of the eSignature REST API.
String accountId = '2ec17bee-90f1-xxxx-af85-b1ee3558f9xx'; String envelopeId = '7c8b166e-37f0-xxxx-b3c8-f80fc5865cxx'; // Create a new http object to send the request object HttpRequest req = new HttpRequest(); // set endpoit to callout:My_Named_Credential/some_path req.setEndpoint('callout:DocusignAPI/accounts/'+accountId+'/envelopes/'+ envelopeId + '/recipients'); req.setMethod('PUT'); req.setHeader('Content-Type', 'application/json'); // Construct the payload Map<String, Object> recipientUpdate = new Map<String, Object>(); recipientUpdate.put('name', 'Mary Doe'); recipientUpdate.put('recipientId', '1'); Map<String,Object> signers = new Map<String,Object>{'signers'=> new List<Map<String,Object>>{recipientUpdate}}; // Convert the payload to JSON String requestBody = JSON.serialize(signers); req.setBody(requestBody); // Perform the callout Http http = new Http(); HttpResponse res = http.send(req); if (res.getStatusCode() == 200) { System.debug('Recipient updated successfully: ' + res.getBody()); } else { System.debug('Error: ' + res.getBody()); }
Step 6: Handle access token expiration
Salesforce handles token refresh automatically through the named credential. You don’t need to manage token renewal manually as long as the auth provider and named credential are set up correctly.
Step 7: Extend and customize
Explore the Docusign eSignature REST API documentation to expand functionality for:
Additional resources
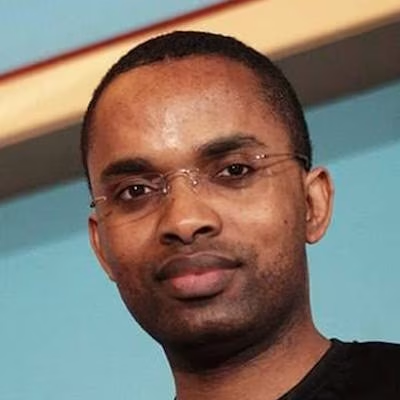
Achille joined Docusign in March 2024. He's a skilled software developer and support engineer with past experience at Google and Salesforce.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
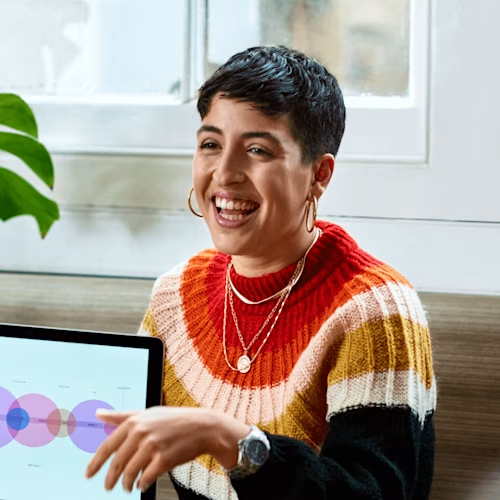